安装RabbitMQ
默认rabbit的命令会被安装到/usr/local/sbin下,这个路径默认不在PATH中,你需要手动添加一下。
启动rabbitmq的方法有两种,一种是使用brew命令:
1
| $ brew services start rabbitmq
|
或者手动启动
默认rabbitmq会建立一个guest用户,密码也是guest,这个用户只能从localhost访问。这个对于我们用于测试学习是够了,如果要建立可以被远程访问的rabbitmq,可以参考access control。
可以访问rabbitmq自带的管理后台查看其运行状态:
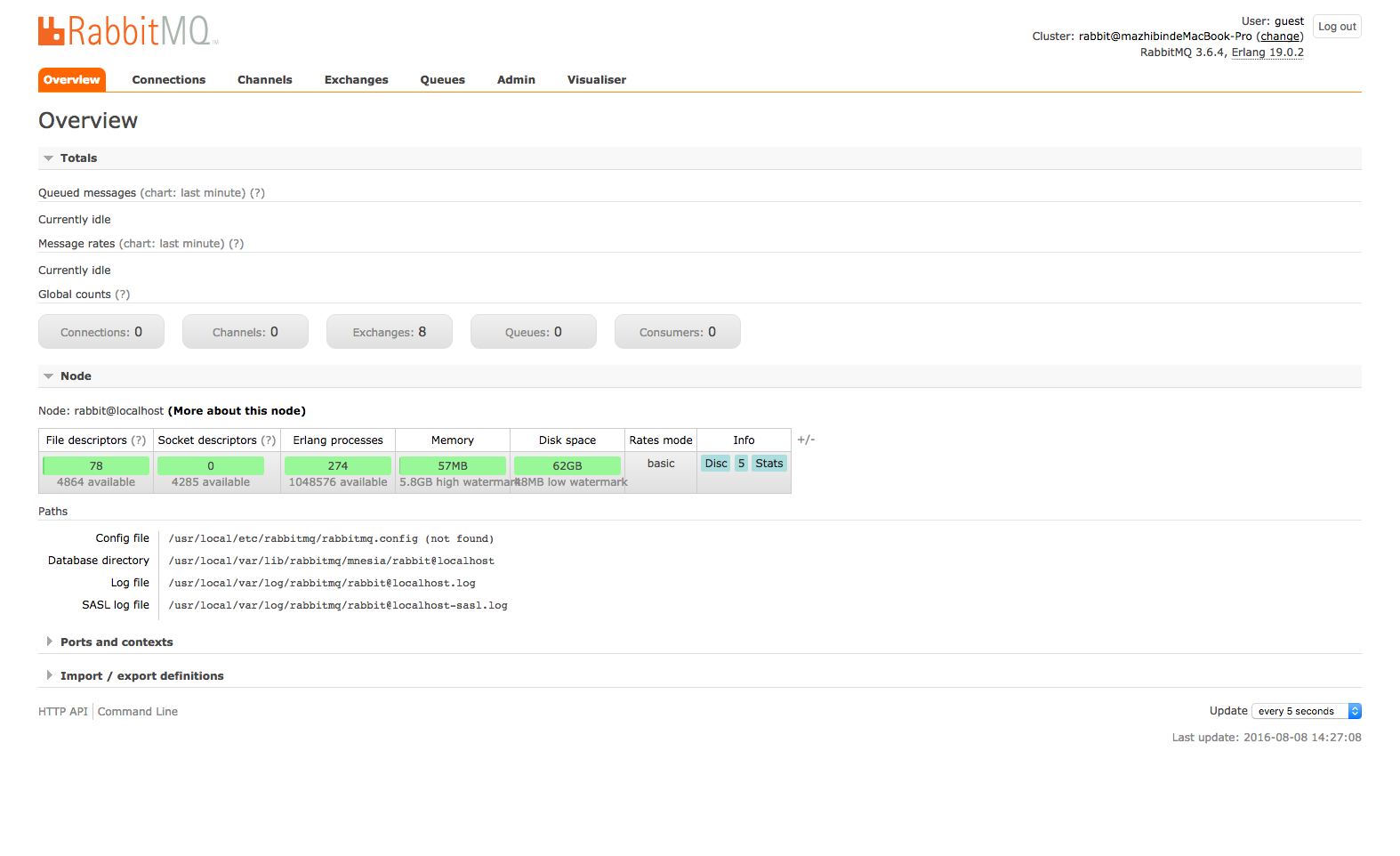
Java使用RabbitMQ
添加依赖
1 2 3 4 5
| <dependency> <groupId>com.rabbitmq</groupId> <artifactId>amqp-client</artifactId> <version>3.6.5</version> </dependency>
|
编写发送方
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| public class Send { public static final String QUEUE_NAME = "hello";
public static void main(String[] args) throws IOException, TimeoutException {
//建立服务器连接,获取通道 ConnectionFactory factory = new ConnectionFactory(); factory.setHost("localhost"); Connection connection = factory.newConnection(); Channel channel = connection.createChannel();
//声明一个发送队列 //声明一个队列是幂等的,仅仅在要声明的队列不存在时才创建 channel.queueDeclare(QUEUE_NAME,false,false,false,null); String msg = "Hello World!"; channel.basicPublish("",QUEUE_NAME,null,msg.getBytes()); System.out.println("Sent msg: "+msg);
//关闭通道和连接 channel.close(); connection.close(); } }
|
编写接收方
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| public class Recv { public static final String QUEUE_NAME = "hello";
public static void main(String[] args) throws IOException, TimeoutException, InterruptedException { //建立服务器连接,获取通道 ConnectionFactory factory = new ConnectionFactory(); factory.setHost("localhost"); Connection connection = factory.newConnection(); Channel channel = connection.createChannel();
//声明一个队列 channel.queueDeclare(QUEUE_NAME,false,false,false,null); System.out.println("Waiting for message...");
//定义一个消费者 QueueingConsumer consumer = new QueueingConsumer(channel); channel.basicConsume(QUEUE_NAME,true,consumer);
//从队列中获取消息并消费 while (true){ QueueingConsumer.Delivery delivery = consumer.nextDelivery(); String msg = new String(delivery.getBody()); System.out.println("Received msg: "+msg); } } }
|
继续学习
RabbitMQ官网的教程写得非常好,图文并茂,推荐阅读:RabbitMQ - Getting started with RabbitMQ
RabbitMQ技巧
在命令行中查看队列消息
1
| $ rabbitmqadmin get queue=hello requeue=false
|
参考:RabbitMQ - Management Command Line Tool
使用rabbitmq_tracing插件记录消息收发日志
1
| $ rabbitmq-plugins enable rabbitmq_tracing
|
然后RabbitMQ Management管理后台中就可以看日志了:

(图片引用自,http://www.cnblogs.com/gossip/p/4517345.html)
参考资料